JavaScript discovery
Processing with AI
Before diving into the world of AI, read the following page.
This are the basics of JavaScript you will need to complete the assignments of Processing with AI.
Good to know
Programming is the process of designing a computer program for accomplishing a specific computing task. It generally involves coding in a specific programming language by writing down lines of code. Don't mix up programming and development, which rather refers to the larger process of testing, debugging (removing bugs), documenting code, managing the program's environment, and so on.
Many programming languages exist for different purposes and personal preferences of developers. The most famous ones include Python, C (along with C++ and C#), Java, PHP, and many more. JavaScript is one of the three languages of the web with HTML and CSS, and therefore can be executed by any browser. Watch out, HTML and CSS are not programming languages but respectively markup and style sheet languages.
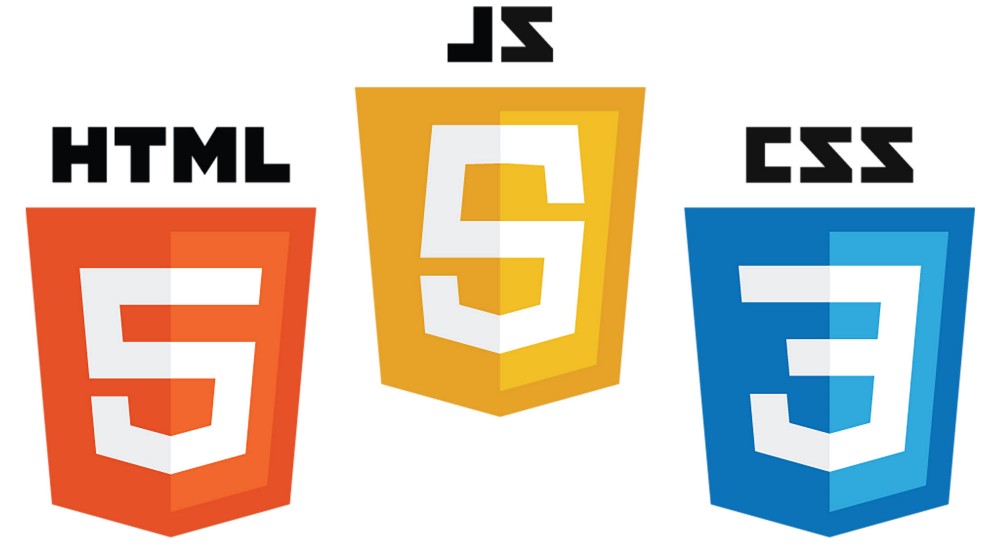
A JavaScript library is a file (or a set of multiple files) of pre-written JavaScript, which allows for easier development of JavaScript-based applications. It allows you to write down working code quicker, starting with a basis that you pick depending on your needs. Basically, a library consists in a set of helpful pre-defined functions and variables that you can use on-the-fly without having to build them from scratch, like p5.js (that we will discover in the next chapter).
1. Functions
A function is a block of code that defines a small… function! It starts with the keyword function
, followed by its name, two parentheses ()
then two curly-brackets {}
.
function myFunction() {
}
Between these brackets is some code, that will be executed each time this function is called.
Now that our function is defined, we can execute its code by writing its name followed by two parentheses anywhere in our code.
myFunction();
A function can take one or more arguments, they are variables that are passed to the function. In that case, when calling the function we will need to provide the value of these arguments in the parentheses
function print_arguments(argument1, argument2) {
print("print_arguments was called");
print("My argument1 is " + argument1);
print("My argument2 is " + argument2);
}
print_arguments(1, 2);
print_arguments("emlyon", "business school");
See the result
print_arguments was called
My argument1 is 1
My argument2 is 2
print_arguments was called
My argument1 is emlyon
My argument2 is business school
Sometimes, when programmers talk about code, to specify they are talking about a function, they write their name followed by two parentheses.
For example "The function that we just saw, called print_arguments()
, prints three lines in the console".
2. Conditions
if
- else
- else if
conditions are very often used, you will need them to add some interactions:
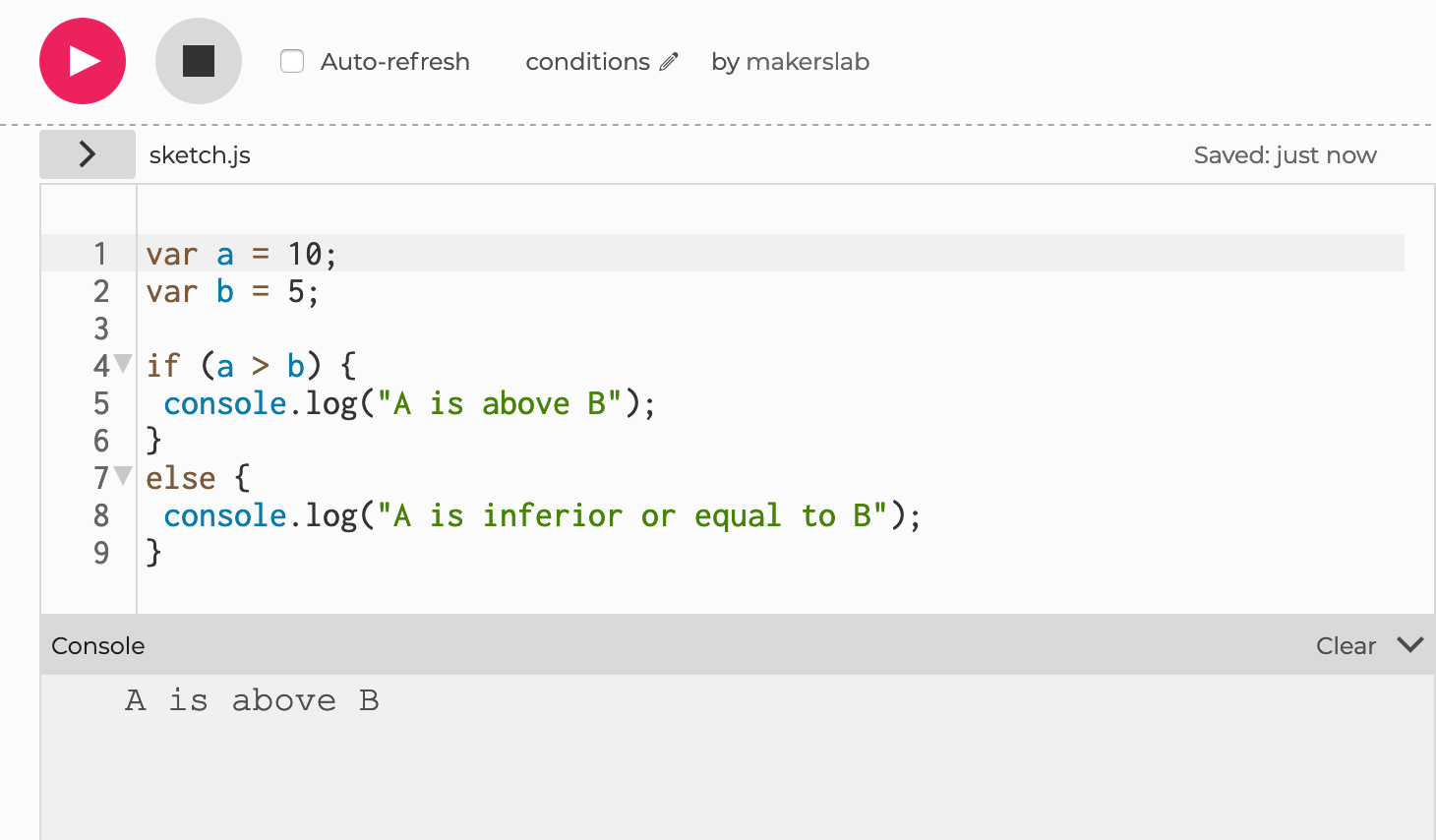
If a
is bigger than b
, write "A is above B" in the console,
else, write "A is inferior or equal to B" in the console".
Have a look at the p5.js reference page about If-Else to learn more about how to use it.
We suggest you watch this video of The Coding Train about all the if-else rules.
p5.js provides a shortcut for console.log()
, a function simply called… print()
3. Logical operators
&&
(and), ||
(or) are two logic operators you can use in your if
- else
- else if
conditions:
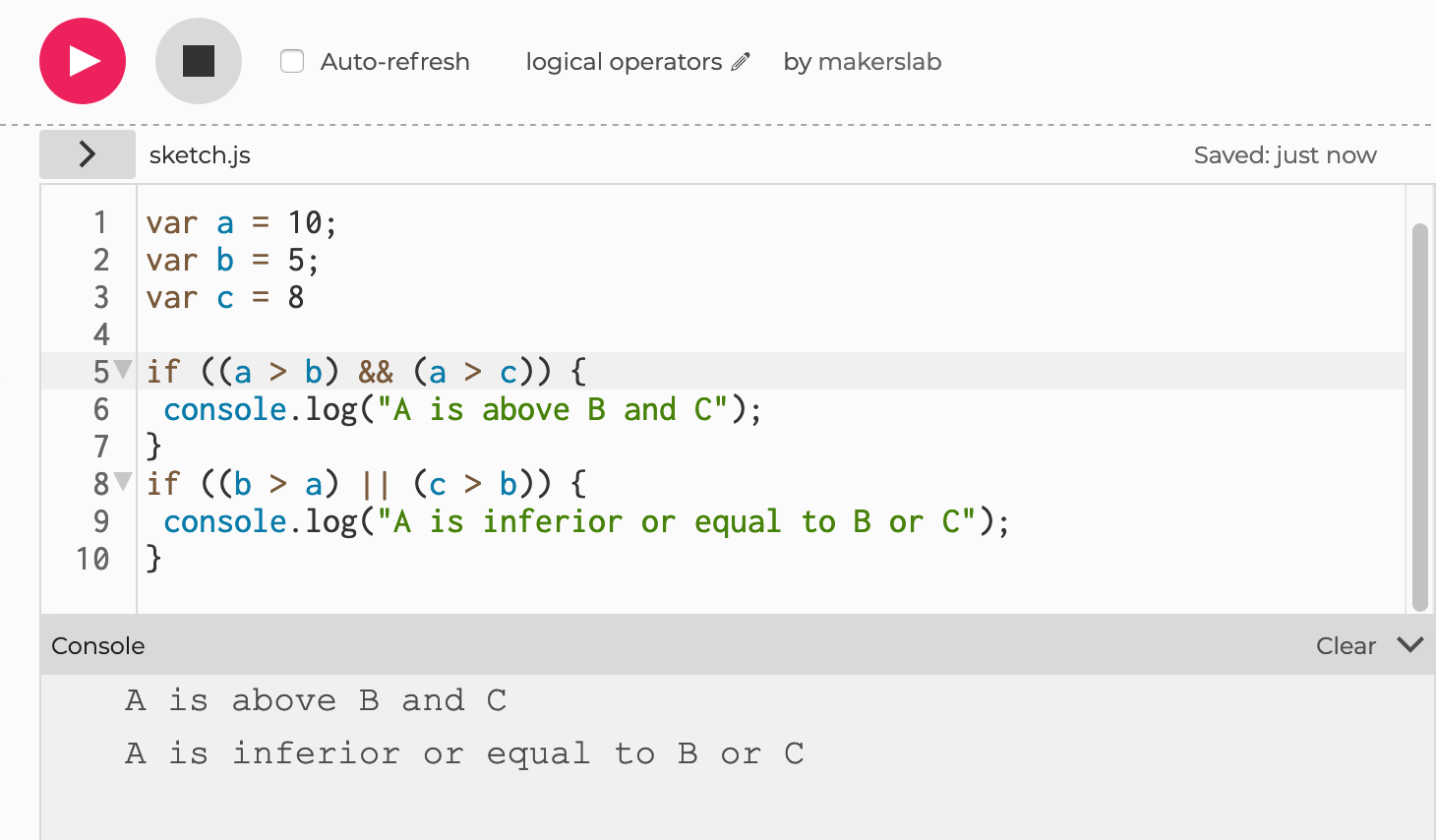
If a
is bigger than b
and a
is bigger than c
write "A is above B and C" in the console
Also, if b
is bigger than a
or c
is bigger than a
write "A is inferior or equal to B or C " in the console.
We suggest you watch this video of The Coding Train about if/else-if and JS conditions.
4. comparison operators
Here are the basic comparison operators you can use in your code:
==
: equal!=
: different>
: greater than>=
: greater or equal to<
: less than<=
: less or equal to
5. Loops
while
and for
are two different kinds of loop useful when a condition is true for multiple values of a variable:
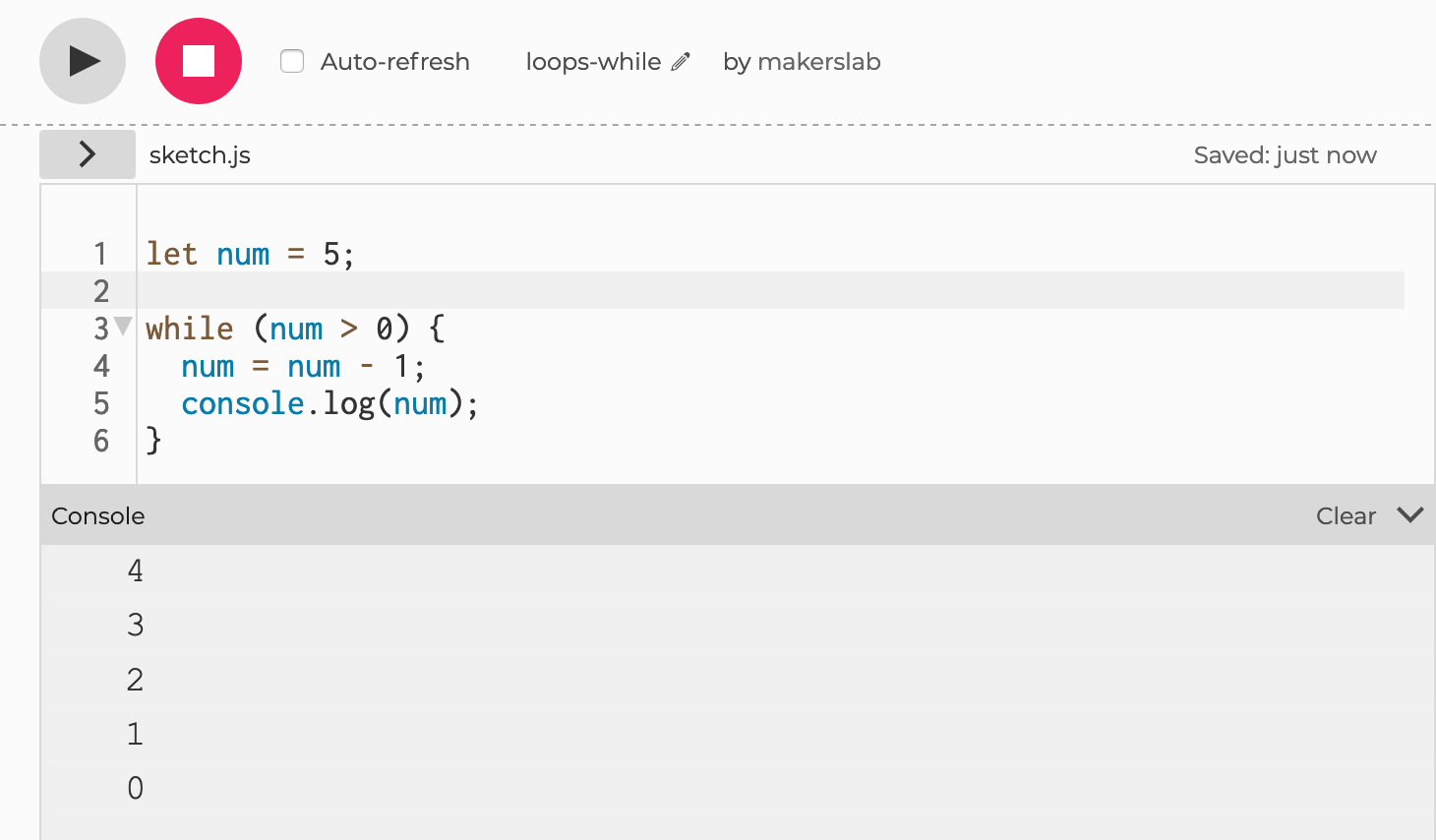
Create a variable called num
and set it to 5
while num
is superior to 0, num
is equal to num
minus 1.
In other words, "while num
is superior to 0 but inferior to 5, num
is equal to itself minus 1, write the value of num
in the console".
Have a look at the p5.js reference page about While loops.
We suggest you watch this video of The Coding Train.
Instead of writing num = num - 1
you can write num--
(there's also num++
)
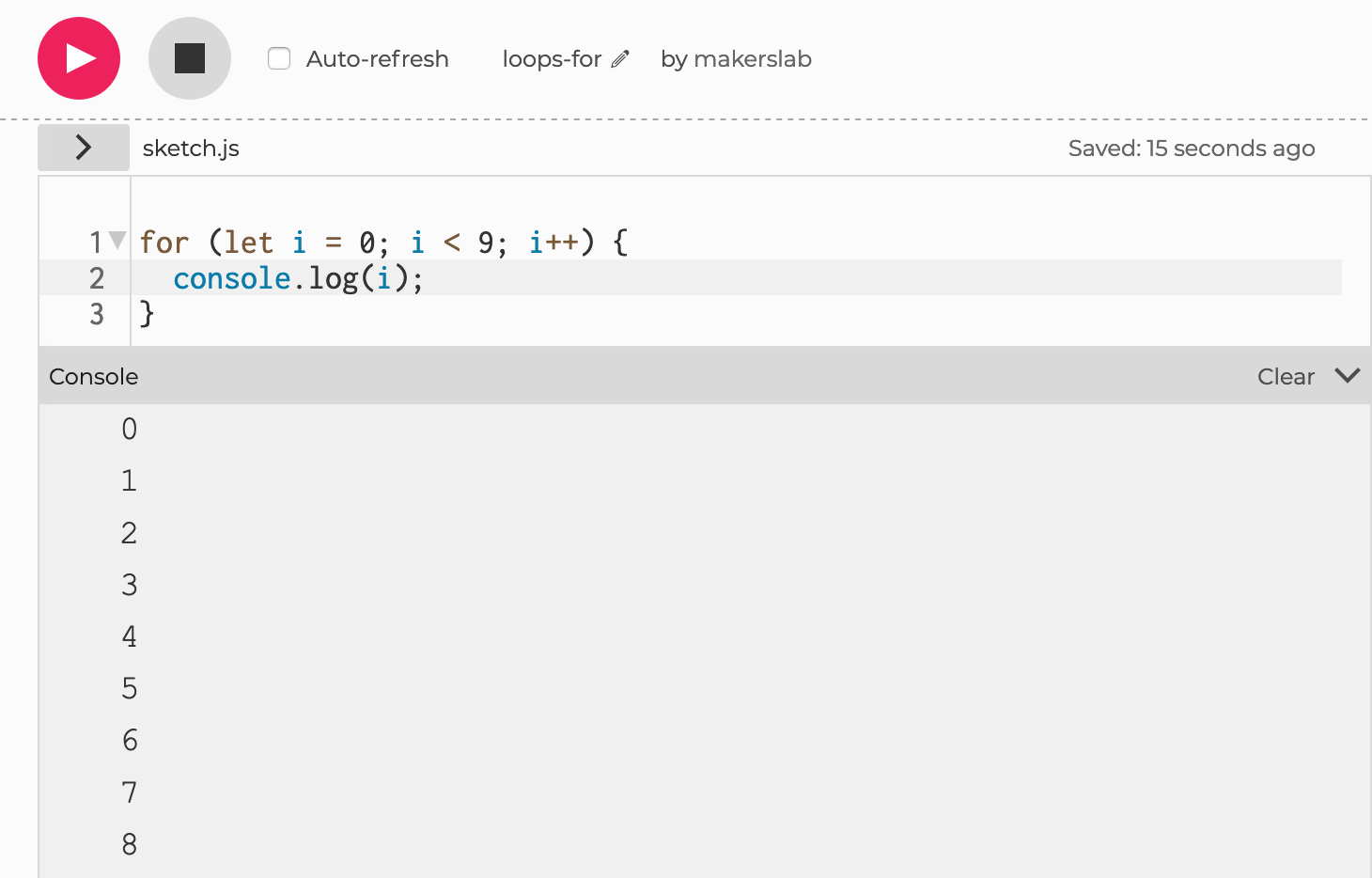
for i
equal to 0 and i
inferior to 9, i
is equal to i
plus 1.
In other words, "when i
is equal to 0, 1, 2, 3, 4, 5, 6, 7, or 8, write the value of i
in the console.
Have a look at the p5.js reference page about for loops.
We suggest you watch this video of The Coding Train.
6. Arrays
arrays
are lists of ordered, stored data. They can hold items that are of any data type and are useful when you need to apply the same function to multiple elements.
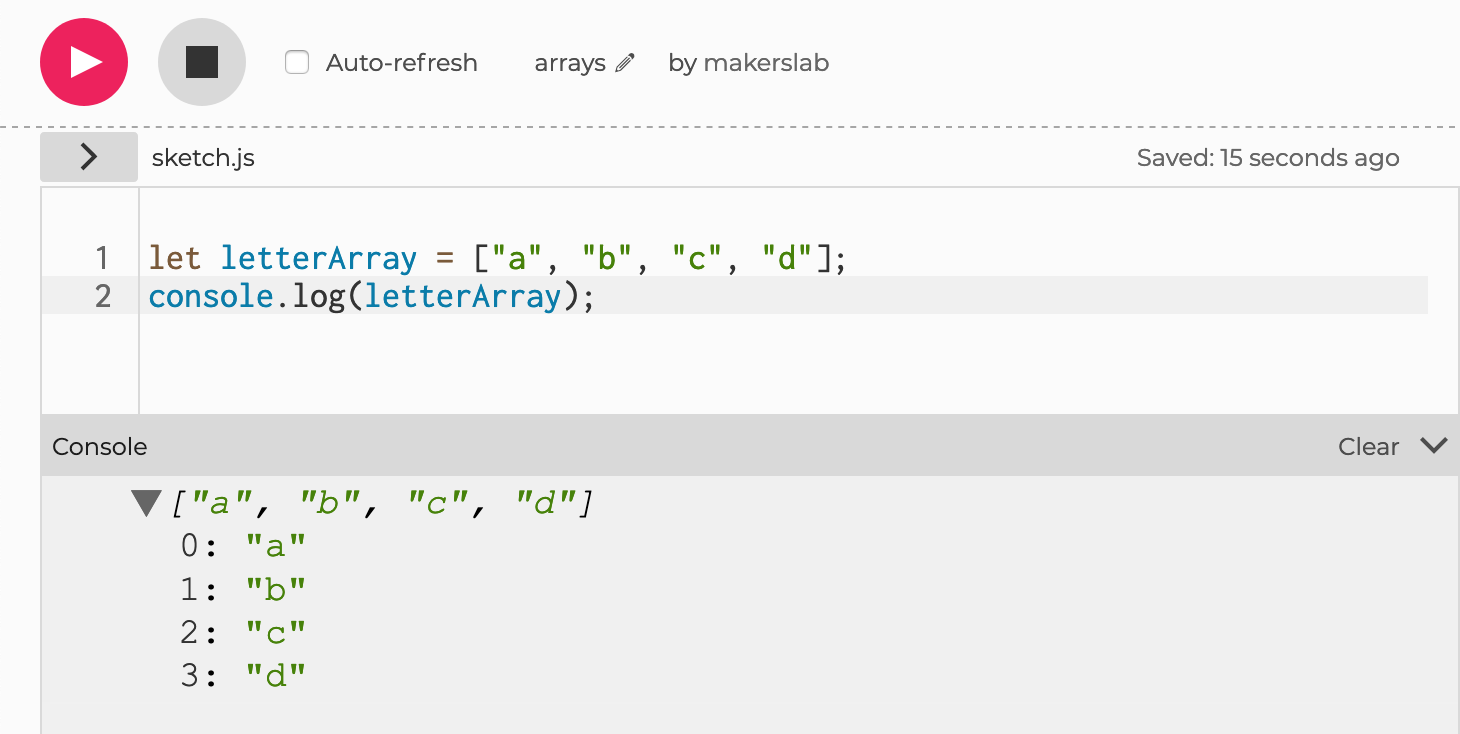
letterArray
is an array of four letters from a to d, write this array in the console.
You can declare an empty array at the beginning of your sketch then fill it later with a for loop for example.
7. Callbacks
Callbacks are used all along this course in the templates. They are functions that are automatically called when a specific event occurs (user clicked on a button, something finished loading, the result of a slow operation is available, etc.). We will use them a lot when implementing the libraries we are going to use.
p5.js, the library we will use in this course has a function named .mousePressed()
that can be used to define a callback on an element. This callback will be called each time we click on the element:
function setup() {
var button = createButton("export");
button.mousePressed(saveImage);
}
function saveImage() {
saveCanvas("myAwesomeDrawing.png");
}
When my user clicks on the button, a function, called saveImage()
will run.
In our case, when the "export" button is pressed, the canvas is saved.
We suggest you watch this very clear video to help you fully understand how callbacks work.
8. Comments
To write a comment in your JavaScript code, use two forward slashes (//
) like shown below. Commented lines will not be executed by the JavaScript engine (it ignores them), which means it's also a great way to temporarily disable a chunk of code without having to entirely delete it.
// comments starts with two forward slashes (//)
function setup() {
// create our canvas element
createCanvas(400, 300);
}
function draw() {
// draw some shapes
// rect(mouseX, mouseY, 20, 20);
ellipse(mouseX, mouseY, random(10, 50));
}
You can also use /*
and */
to define a comment block.
function test() {
/* This is a
multi-line
comment */
}
9. Debugging
When an error occurs in your code, you can consult the console to find out what happens and get more infos to correct it:
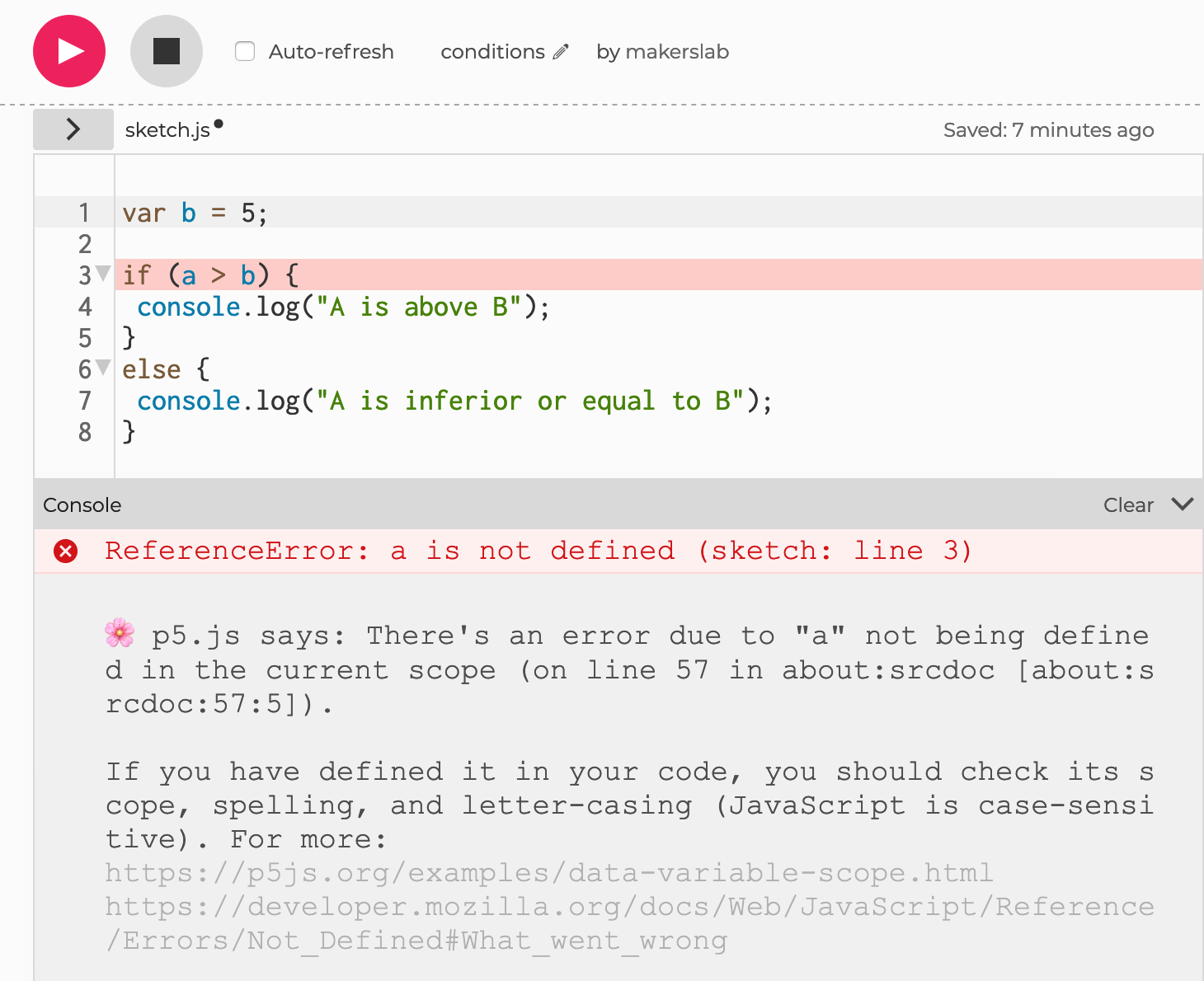
The highlighted line in red is where the error occurs. We can read in the console that the error is due to our variable a
which is undefined yet.
We have to define it before using it (with var a = 10;
for example) to fix this problem.
As you might remember from Designing with Web, your browser also have a JS console to test code / print informations and errors for developers:
- Chrome: press
Ctrl
+Shift
+J
(Windows) /Cmd
+Opt
+J
(Mac) - Firefox: press
Ctrl
+Shift
+K
(Windows) /Cmd
+Opt
+K
(Mac)
These more advanced consoles will often give you more information than the one in the p5.js editor.
Going further
JavaScript was created in 1995 at Netscape, a very popular web browser back in the 90s. In the beginning, it only had client-side uses, which means it was only exectued by one's browser. However, the next year, Netscape introduced an implementation of the language for server-side scripting. Nowadays, many more implementations have been introduced, such as the widely-used Node.js.
Have a look at this JavaScript Cheat Sheet for a recap of this page along with other quick JS tips!
A word about text editors
Unlike what is shown in this module, programming is usually done using an IDE or a text editor, which is a computer program that edits plain text. Unlike other text editors like Word, IDE allows programmers to code more efficiently thanks to features like syntax highlighting, code completion, linter, etc. If you want to learn more about IDE advantages, read this Wikipedia page.
Here's a list of some famous IDE:
- Visual Studio Code, a free and powerful text editor
- Atom, another free and customizable text editor
- Sublime Text, a fully customizable text editor (have a look at Package Control for a list of available plugins!)
In this course, you will mainly use an online IDE provided by p5.js (the screenshots on this page were all done using it).
Continue by reading the next chapter 🚀 Starting with p5.js to learn how to use it!